ThinkPHP5.0.24初学
ThinkPHP 5.0.24
1、路由分析
- 入口文件:Public/index.php
- 和TP3版本不一样的地方
- 入口不同
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006-2016 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <[email protected]>
// +----------------------------------------------------------------------
// [ 应用入口文件 ]
// 定义应用目录
define('APP_PATH', __DIR__ . '/../application/');
// 加载框架引导文件
require __DIR__ . '/../thinkphp/start.php';
2、自定义入口应用目录
-
复制application目录,重命名为Home目录,并修改入口文件。
-
index.php
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006-2016 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <[email protected]>
// +----------------------------------------------------------------------
// [ 应用入口文件 ]
// 定义应用目录
define('APP_PATH', __DIR__ . '/../Home');
// 加载框架引导文件
require __DIR__ . '/../thinkphp/start.php';
- 修改Home/Index/controller/index.php
<?php
namespace app\index\controller;
class Index
{
public function index()
{
echo "Hello TP5";
}
// 自定义方法
public function haha(){
echo "HAHA";
}
}
- 访问前台
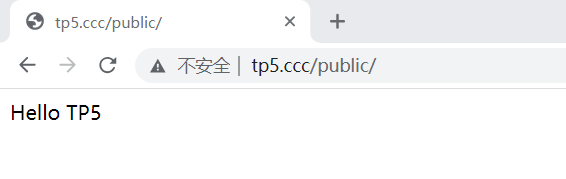
- 访问自定义方法
http://tp5.ccc/public/index.php/index/index/haha
不在支持TP3这种写法
http://tp5.ccc/public/index.php?m=index&c=index&a=haha
-
自定义新的控制器模块
-
复制Home/index目录,重命名为Admin,修改index.php
<?php
namespace app\admin\controller;
class Index
{
public function index()
{
echo "欢迎!管理员";
}
// 自定义方法
public function haha(){
echo "HAHA";
}
}
- 前台访问
http://tp5.ccc/public/index.php/admin/index/
3、模板的使用
- 在Home\index\下创建index\view目录,并创建一些html文件
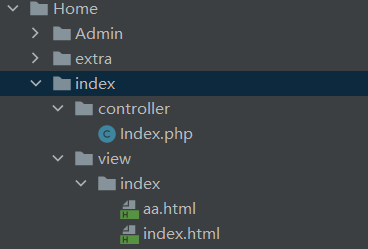
- 修改index.php
<?php
namespace app\index\controller;
use think\Controller;
class Index extends Controller
{
public function index()
{
// tp5推荐使用return
return $this->fetch("index");
}
// 自定义方法
public function haha(){
echo "HAHA";
}
}
- 前台访问
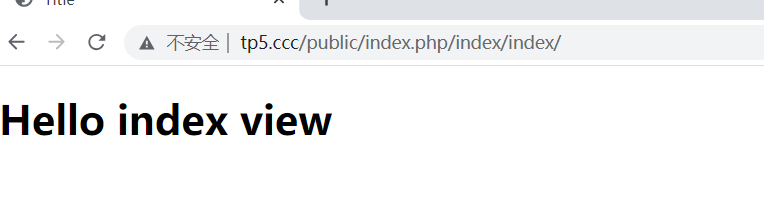
4、留言板搭建
- 定义不功能的函数
<?php
namespace app\index\controller;
use think\Controller;
class Index extends Controller
{
// 首页
public function index()
{
// tp5推荐使用return
return $this->fetch("index");
}
// 编辑留言
public function edit(){
return $this->fetch();
}
// 发布留言
public function pub(){
return $this->fetch();
}
// 管理留言
public function manage(){
return $this->fetch();
}
}
4.1、ROOT关键字
- TP5用__ROOT关键字来替代TP3中的\PUBLIC__关键字,用来定位到public目录
4.2、定义前台js模板常量
- 在/Home/index/目录下创建一个config.php文件
<?php
return [
// 视图输出字符串内容替换
'view_replace_str' => [
// 可以通过这种方法来定义会PUBLIC关键字
"__PUBLIC__" =>"/public/static",
],
];
- 在public/static/目录下创建index目录,把样式文件放进入,并修改html的代码
4.3、修改header.html
- 因为tp5有些函数已经不支持或者修改了名字
tp5变成url 而不在是U
:url('index/Index/index')
- 修改后
<!--<!DOCTYPE unspecified PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">-->
<html><head><meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta http-equiv="Cache-Control" content="no-transform">
<title>简易留言板</title>
<meta content="简易、留言板、THINKPHP、入门" name="keywords">
<meta content="新手可以看的简易留言板" name="description">
<link href="__PUBLIC__/Index/default.css" media="screen" rel="stylesheet" type="text/css">
<script src="__PUBLIC__/Index/jquery.js" type="text/javascript"></script>
</head>
<body>
<div id="header">
<div class="logo"><a href="{:url('index/Index/index')}">LYB</a></div>
<div class="headerLink">
<a href="{:url('index/Index/index')}">首页</a>
<a href="{:url('index/Index/pub')}">发布留言</a>
<a href="{:url('index/Index/manage')}">管理留言</a>
</div>
</div>
4.4、连接数据库
- 修改Home/database.php
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006~2018 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <[email protected]>
// +----------------------------------------------------------------------
return [
// 数据库类型
'type' => 'mysql',
// 服务器地址
'hostname' => '127.0.0.1',
// 数据库名
'database' => 'thinkphp33',
// 用户名
'username' => 'root',
// 密码
'password' => 'root',
// 端口
'hostport' => '3306',
// 连接dsn
'dsn' => '',
// 数据库连接参数
'params' => [],
// 数据库编码默认采用utf8
'charset' => 'utf8',
// 数据库表前缀
'prefix' => '',
// 数据库调试模式
'debug' => true,
// 数据库部署方式:0 集中式(单一服务器),1 分布式(主从服务器)
'deploy' => 0,
// 数据库读写是否分离 主从式有效
'rw_separate' => false,
// 读写分离后 主服务器数量
'master_num' => 1,
// 指定从服务器序号
'slave_no' => '',
// 自动读取主库数据
'read_master' => false,
// 是否严格检查字段是否存在
'fields_strict' => true,
// 数据集返回类型
'resultset_type' => 'array',
// 自动写入时间戳字段
'auto_timestamp' => false,
// 时间字段取出后的默认时间格式
'datetime_format' => 'Y-m-d H:i:s',
// 是否需要进行SQL性能分析
'sql_explain' => false,
];
- 测试连接是否正常,已经变成db来调用
<?php
namespace app\index\controller;
use think\Controller;
class Index extends Controller
{
// 首页
public function index()
{
// tp5推荐使用return
// return $this->fetch();
// 测试连接数据库
$collection = db("Comment")->select();
dump($collection);
}
}
4.5、定义分页显示
- 在config.php中定义分页常量
<?php
return [
// 视图输出字符串内容替换
'view_replace_str' => [
// 可以通过这种方法来定义会PUBLIC关键字
"__PUBLIC__" =>"/public/static",
],
// 定义分页常量
'PAGE_LIMIT' =>10,
];
- 修改index.php
// 首页
public function index()
{
// 调用分页常量
$limit = config("PAGE_LIMIT");
// 查询状态为1的用户数据 并且每页显示10条数据
$list = db("Comment")->order("id desc")->paginate($limit);
// 获取分页显示
$page = $list->render();
// 模板变量赋值
$this->assign('list', $list);
$this->assign('page', $page);
// 渲染模板输出
return $this->fetch();
}
- 修改index.html
<include file="common/header" />
<div id="main">
<script type="text/javascript">
$(function(){
$(".headerLink a:eq(0)").addClass('headerLinkSelect');
});
</script>
<table>
<tbody><tr class="listtr">
<th class="listth" style="width: 10%;">留言IP</th>
<th class="listth">留言主题</th>
<th class="listth">留言内容</th>
<th class="listth" style="width: 18%;">留言时间</th>
</tr>
{volist name='list' id='user'}
<tr class="listtr" style="line-height:32px;">
<td class="listtd alignCenter">{$user.ip}</td>
<td class="listtd">{$user.title}</td>
<td class="listtd">{$user.content}</td>
<td class="listtd alignCenter">{$user.time}</td>
</tr>
{/volist}
<tr class="listtr">
<td class="listtd" style="background-color: #f0f0f0;" colspan="4">
<div>
{$page}</div>
</td>
</tr>
</tbody></table>
</div>
</body>
</html>
4.6、发布留言
- 把自定义的函数放到公共函数中,Home\common.php
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006-2016 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: 流年 <[email protected]>
// +----------------------------------------------------------------------
// 应用公共文件
function get_client_ip($type = 0)
{
$type = $type ? 1 : 0;
static $ip = NULL;
if ($ip !== NULL) {
return $ip[$type];
}
if (isset($_SERVER['HTTP_X_REAL_IP'])) {
//nginx 代理模式下,获取客户端真实IP
$ip = $_SERVER['HTTP_X_REAL_IP'];
} elseif (isset($_SERVER['HTTP_CLIENT_IP'])) {
//客户端的ip
$ip = $_SERVER['HTTP_CLIENT_IP'];
} elseif (isset($_SERVER['HTTP_X_FORWARDED_FOR'])) {
//浏览当前页面的用户计算机的网关
$arr = explode(',', $_SERVER['HTTP_X_FORWARDED_FOR']);
$pos = array_search('unknown', $arr);
if (false !== $pos) unset($arr[$pos]);
$ip = trim($arr[0]);
} elseif (isset($_SERVER['REMOTE_ADDR'])) {
//浏览当前页面的用户计算机的ip地址
$ip = $_SERVER['REMOTE_ADDR'];
} else {
$ip = $_SERVER['REMOTE_ADDR'];
}
// IP地址合法验证
$long = sprintf("%u", ip2long($ip));
$ip = $long ? array($ip, $long) : array('0.0.0.0', 0);
return $ip[$type];
}
- 编写pub
// 发布留言
public function pub(){
//获取IP
$ip = get_client_ip();
if (!empty(input("submitLy"))){
$ip = input("ip");
$title = input("title");
$content = input("content");
$time = date("Y-m-d H:i:s",time());
$data = [
"ip"=>$ip,
"title"=>$title,
"content"=>$content,
"time"=>$time
];
$result = db("Comment")->insert($data);
$result ===1?$this->success("留言成功",url('index/Index/index'),3):$this->error("留言失败");
}else{
$this->assign('ip',$ip);
return $this->fetch();
}
}
4.7、管理留言
- 修改manage.html
<include file="common/header" />
<div id="main">
<script type="text/javascript">
$(function(){
$(".headerLink a:eq(2)").addClass('headerLinkSelect');
});
</script>
<table>
<tbody><tr class="listtr">
<th class="listth" style="width: 10%;">留言IP</th>
<th class="listth">留言主题</th>
<th class="listth">留言内容</th>
<th class="listth" style="width: 18%;">留言时间</th>
<th class="listth" style="width: 10%;">操作</th>
</tr>
{volist name='list' id='vo'}
<tr class="listtr" style="line-height:32px;">
<td class="listtd alignCenter">{$vo.ip}</td>
<td class="listtd">{$vo.title}</td>
<td class="listtd">{$vo.content}</td>
<td class="listtd alignCenter">{$vo.time}</td>
<td class="listtd alignCenter">
<a href="{:url('index/index/edit',['id'=>$vo['id']])}">修改</a>
<a href="{:url('index/index/del',['id'=>$vo['id']])}">删除</a></td>
</tr>
{/volist}
<tr class="listtr">
<td class="listtd" style="background-color: #f0f0f0;" colspan="5">
<div> {$page} </div>
</td>
</tr>
</tbody></table>
</div>
</body></html>
- 编写manage.php
public function manage(){
// 调用分页常量
$limit = config("PAGE_LIMIT");
// 查询状态为1的用户数据 并且每页显示10条数据
$list = db("Comment")->order("id desc")->paginate($limit);
// 获取分页显示
$page = $list->render();
// 模板变量赋值
$this->assign('list', $list);
$this->assign('page', $page);
return $this->fetch();
}
4.8、修改留言
public function edit(){
$ip = get_client_ip();
if (!empty(input('submitLy'))){
$id = input("id");
$ip = input("ip");
$title = input("title");
$content = input("content");
$time = date("Y-m-d H:i:s",time());
$data = [
"ip"=>$ip,
"title"=>$title,
"content"=>$content,
"time"=>$time
];
$result = db("Comment")->where("id",$id)->update($data);
$result ===1?$this->success("修改成功",url('index/Index/manage'),3):$this->error("修改失败");
}else{
$this->assign('ip',$ip);
$id = input("id");
$data = db("comment")->where("id",$id)->find();
$this->assign('ip',$ip);
$this->assign('data',$data);
return $this->fetch();
}
}
4.8、删除留言
// 删除留言
public function del(){
if (!empty(input("id"))){
$id = input("id");
$result = db('comment')->where("id", $id)->delete();
$result ===1?$this->success("删除成功",url('index/Index/manage'),3):$this->error("删除失败");
}else{
$this->error("删除失败",url('index/Index/manage'),3);
}
}